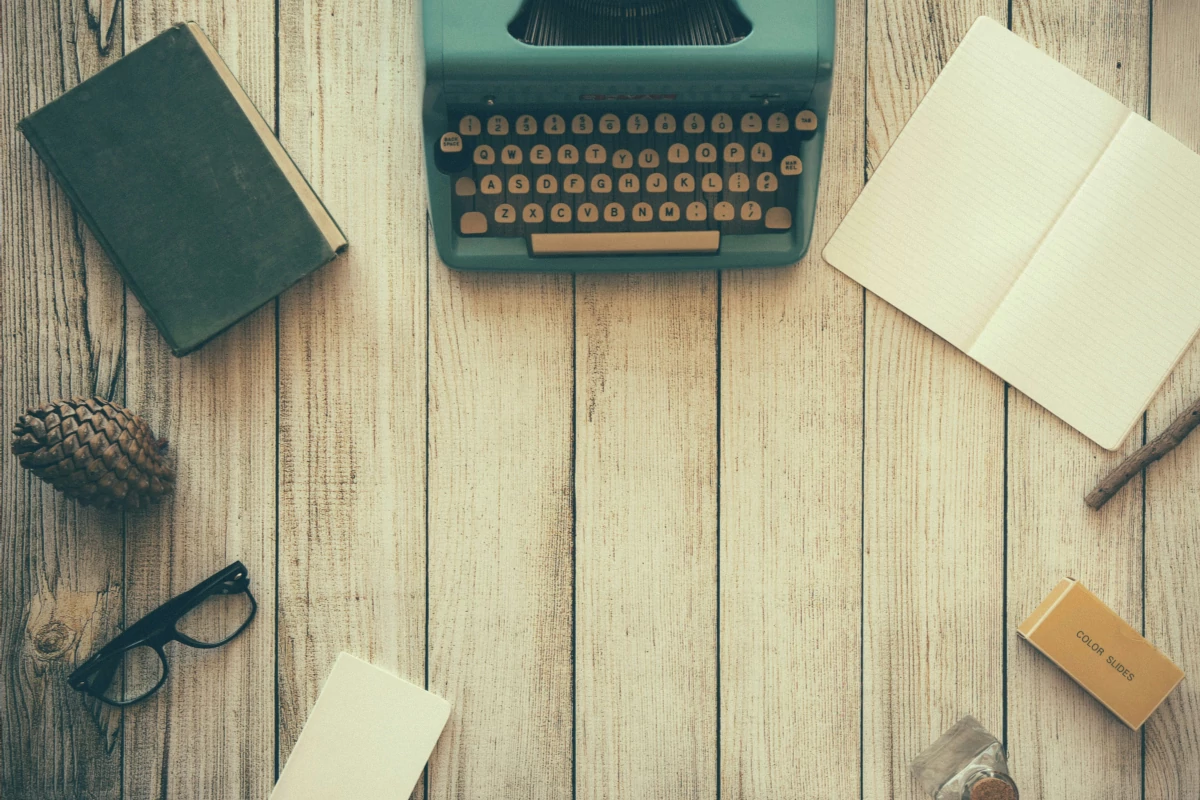
Learn to search comma-separated fields in MySQL databases using FIND_IN_SET(). Perfect for developers working with non-normalized data structures.
FIND_IN_SET()
When working with databases, it's best practice to normalize your data — especially when storing multiple values. However, in real-world scenarios (like legacy systems or quick projects), developers often store multiple values in a comma-separated format in a single field.
In this blog, we'll show you how to extract specific data from such fields using MySQL's built-in function FIND_IN_SET()
with a practical example using PHP and MySQLi.
Let's say you have a table named doctorsdb_doctors
with a structure like this:
doctorsid | doctors_name | doctors_specilization |
23 | Dr. Sunit Upadhye | Pediatric Care,Diabetic Care,Acne |
33 | Dr. Aakash Chavhan | Pediatric Care,Allergic Rhinitis |
41 | Dr. Ravi Patil | Diabetic Care,Hypertension |
You want to fetch all doctors who offer Pediatric Care.
Here's how to use FIND_IN_SET()
in your SQL:
This query will return any row where 'Pediatric Care' exists as a distinct value in the doctors_specilization
field, regardless of its position.
Here’s how you can run this query in PHP using MySQLi:
FIND_IN_SET()
is a handy MySQL function to search for a value in a comma-separated list.(2) (0)
No comments